Tailwind CSS with Parcel v2
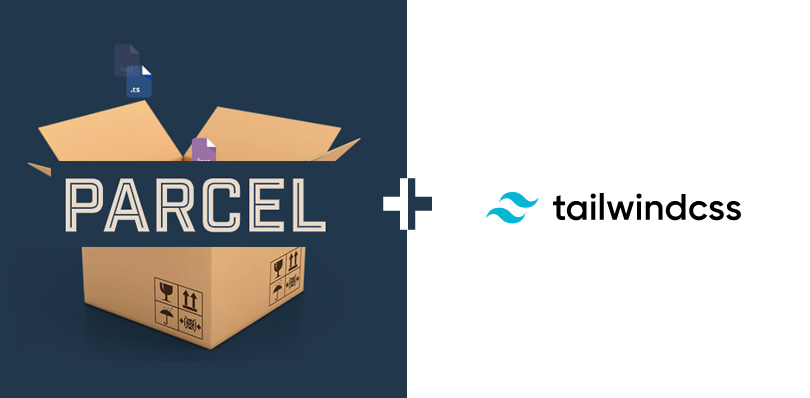
Getting Tailwind to compile with Parcel v2 is easier than ever. We will leverage the Parcel v2 PostCSS support to automatically compile Tailwind assets with little configuration.
I assume that you use yarn for all node packages. If you use npm please use the equivalent commands.
Start by initializing your project with:
yarn init
Then add the required dependencies:
yarn add -D tailwindcss@latest postcss parcel@next cssnano autoprefixer@latest postcss-import
This will install:
- Tailwind CSS: the actual framework itself
- PostCSS: the CSS post processor that will compile the Tailwind assets into CSS
- Parcel: the web app bundler
- CSSNano: CSS minifier
- Autoprefixer: add vendor prefixes to fancy CSS
- PostCSS Import: plugin that allows you to import CSS files into other CSS files
Now, there are a couple of things you will need to create:
- A .postcssrc file: which will contain the PostCSS settings
- A tailwind.config.js file which will contain the Tailwind CSS settings (like themes, colors, etc)
- A .css file which will import all the Tailwind libraries (think of it as your CSS entrypoint)
Start by creating a .postcssrc
file in the root folder of your project and add the following contents to it:
// ./.postcssrc
{
"plugins": {
"postcss-import": {},
"tailwindcss/nesting": {},
"tailwindcss": {},
"autoprefixer": {},
"cssnano": {}
}
}
Then create a tailwind.config.js
file in the root folder of your project and add the following contents to it:
/* ./tailwind.config.js */
module.exports = {
mode: "jit",
purge: [],
darkMode: false, // or 'media' or 'class'
theme: {},
variants: {},
plugins: [],
};
Important: the “purge” array should contain the relative path to your HTML templates. This is especially important for your production environment so your resulting CSS is as light as possible. PostCSS will read those files, scan for classes that are actually in use and only compile those. All unused classed will not be compiled, which will give you a well optimized CSS file. The following example should get you started:
purge: [
'./src/**/*.html',
'./src/**/*.js',
],
Bonus: if you are using Laravel, use the following “purge” settings:
purge: [
'./resources/**/*.blade.php',
'./resources/**/*.js',
'./resources/**/*.vue',
],
Next, create your entrypoint .css
file, which you can call style.css and place it wherever your resources should go. Then add the following to it:
/* ./your-css-folder/styles.css */
@import "tailwindcss/base";
@import "tailwindcss/components";
@import "tailwindcss/utilities";
Now comes the interesting part. We need to tell Parcel where to go to compile your CSS. To do this, we will add our scripts to package.json
like so:
"scripts": {
"watch-css": "parcel watch resources/css/style.css --dist-dir public/css --public-url /css",
"build-css": "parcel build resources/css/style.css --dist-dir public/css --public-url /css --no-hmr",
"production-css": "NODE_ENV=production parcel build resources/css/style.css --dist-dir public/css --public-url /css --no-content-hash --no-source-maps"
},
The paths in the scripts section of your package.json depend on your project, so make sure you update those.
Then simply run:
yarn watch-css
yarn build-css
yarn production-css
…depending on the environment.
As always, have a look around in the official Tailwind CSS docs for additional settings and customization.