Laravel reCAPTCHA v2 Middleware in 3 minutes
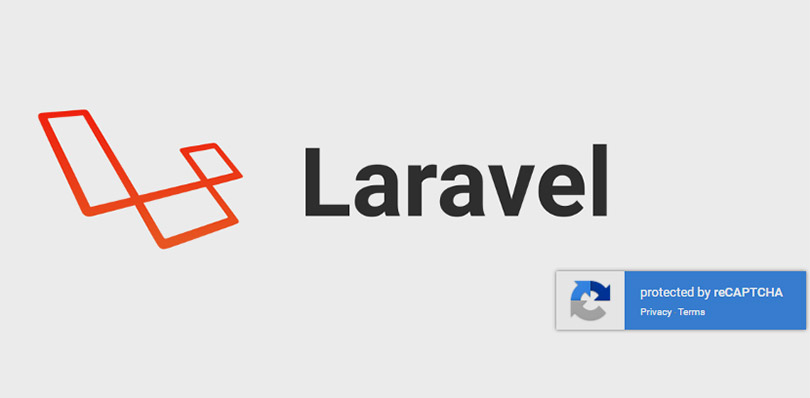
I needed to add reCAPTCHA v2 to a couple of forms and most of the results on Google pointed to these large Composer packages that were simply too much.
This article will cover how to do create a new Laravel Middleware to validate reCAPTCHA v2 requests with just a few lines of code.
Time to read, implement, QA and deploy to production: 3.141592 minutes.
Before you get started, make sure your frontend is ready for reCAPTCHA. This is a mandatory prerequisite for any reCAPTCHA implementation, not just Laravel. Register a new application with reCAPTCHA, get your keys and all that jazz.
Now the fun part: add a couple of ReCaptcha config entries to your .env file:
RECAPTCHA_SITE_KEY=your_recaptcha_site_key
RECAPTCHA_SECRET_KEY=your_recaptcha_secret_key
Make sure the official PHP ReCaptcha library is installed with Composer:
composer require google/recaptcha "^1.2"
Create a new Middleware called “Recaptcha”:
php artisan make:middleware Recaptcha
Edit your new Middleware to look like so:
<?php
namespace App\Http\Middleware;
use Closure;
use ReCaptcha\ReCaptcha as GoogleRecaptcha;
class Recaptcha
{
/**
* Handle an incoming request.
*
* @param \Illuminate\Http\Request $request
* @param \Closure $next
* @return mixed
*/
public function handle($request, Closure $next)
{
$response = (new GoogleRecaptcha(env('RECAPTCHA_SECRET_KEY')))
->verify($request->input('g-recaptcha-response'), $request->ip());
if (!$response->isSuccess()) {
return redirect()->back()->with('status', 'Recaptcha failed. Please try again.');
}
return $next($request);
}
}
Register the Middleware in app/Http/Kernel.php
:
protected $routeMiddleware = [
// ...
'recaptcha' => \App\Http\Middleware\Recaptcha::class,
// ...
];
You’re done!
Now, assign your middleware to your routes:
Route::post('user/signup', function () {
//
})->middleware('recaptcha');
Or to your controllers:
class UserController extends Controller
{
/**
* Instantiate a new controller instance.
*
* @return void
*/
public function __construct()
{
$this->middleware('recaptcha');
}
}
That’s pretty much it. Remember to make sure your frontend is working properly or the middleware won’t do much if nothing at all.
Say adios to those pesky spammers and robots!